Assign Materials to Collection
This script assigns materials from a defined list to mesh objects in a specified collection, cycling through the materials and adding warnings for missing ones.
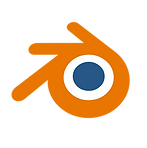
Broken button... I'll fix it eventually...
# Import Blender API
import bpy
# Define the collection name
collection_name = "Your_Collection_Name" # Replace with the name of your collection
# Material names to assign
material_names = ["Material 1", "Material 2", "Material 3"]
# Retrieve the collection
collection = bpy.data.collections.get(collection_name)
if collection:
for i, obj in enumerate(collection.objects):
# Ensure the object is a mesh
if obj.type == 'MESH':
# Add material slots to the object if not present
while len(obj.data.materials) < len(material_names):
obj.data.materials.append(None)
# Assign materials in a cycle
for j, mat_name in enumerate(material_names):
material = bpy.data.materials.get(mat_name)
if material:
obj.data.materials[j] = material
else:
print(f"Material '{mat_name}' not found.")
else:
print(f"Collection '{collection_name}' not found.")
Description
This script assigns specified materials to all mesh objects within a designated collection in Blender. It ensures each object has enough material slots and applies the materials in a cyclic pattern from a provided list. If a material is not found, a warning is printed, allowing users to identify and address missing materials.