Blender - Custom Shader Replaces Image BDSF
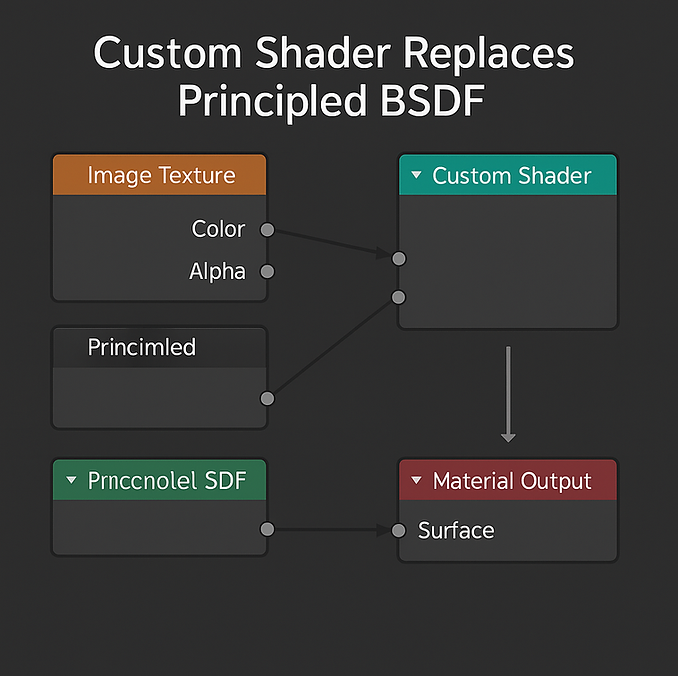
This script inserts a custom node group into the materials of selected objects, connects image texture inputs, and removes the default Principled BSDF node for a cleaner shader setup.
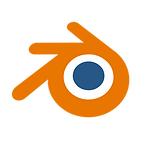
Broken button... I'll fix it eventually...
import bpy
# Name of the custom node group in Blender
custom_node_group_name = "Arrows/Lines" # Replace with the name of your custom node group
# Iterate through selected objects
for obj in bpy.context.selected_objects:
if obj.type == 'MESH' and obj.data.materials: # Ensure the object is a mesh with materials
for material in obj.data.materials:
if material and material.use_nodes: # Ensure the material uses nodes
node_tree = material.node_tree
nodes = node_tree.nodes
# Find the existing image texture node, material output, and principled BSDF
image_texture_node = None
material_output_node = None
principled_bsdf_node = None
for node in nodes:
if node.type == 'TEX_IMAGE':
image_texture_node = node
elif node.type == 'OUTPUT_MATERIAL':
material_output_node = node
elif node.type == 'BSDF_PRINCIPLED':
principled_bsdf_node = node
# If both image texture and material output nodes are found, insert the custom node group
if image_texture_node and material_output_node:
# Add the custom node group
custom_node = nodes.new(type='ShaderNodeGroup')
custom_node.node_tree = bpy.data.node_groups[custom_node_group_name]
# Position the custom node between the image texture and material output
custom_node.location = (
(image_texture_node.location.x + material_output_node.location.x) / 2,
(image_texture_node.location.y + material_output_node.location.y) / 2
)
# Rewire the connections
# Connect the Color output of the Image Texture to the first input of the custom node
node_tree.links.new(image_texture_node.outputs['Color'], custom_node.inputs[0]) # Adjust input index as needed
# Connect the Alpha output of the Image Texture to the second input of the custom node (if applicable)
if 'Alpha' in image_texture_node.outputs:
node_tree.links.new(image_texture_node.outputs['Alpha'], custom_node.inputs[1]) # Adjust input index as needed
# Connect the custom node's output to the Material Output node
node_tree.links.new(custom_node.outputs[0], material_output_node.inputs[0]) # Adjust output index as needed
# Delete the Principled BSDF node if it exists
if principled_bsdf_node:
nodes.remove(principled_bsdf_node)
print("Custom node group inserted, and Principled BSDF node deleted for selected objects successfully.")
Description
This script automates the process of modifying materials for selected objects in Blender by inserting a custom node group into the shader node tree. It connects the color and alpha outputs of the image texture node to the custom node group and links the custom node's output to the material output. Additionally, it cleans up the material by deleting the default Principled BSDF node, ensuring a streamlined and efficient node setup.