Bulk Import - SketchUp .dae to Blender
The SketchUp .dae Importer is a Blender add-on designed for batch importing .dae files exported from SketchUp, organizing them into collections, and cleaning up unnecessary objects. It streamlines workflows by automatically joining geometry, moving extras like curves and empties into separate collections, and eliminating imported cameras.
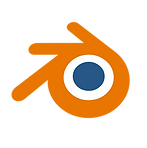
Broken button... I'll fix it eventually...
bl_info = {
"name": "SketchUp .dae Importer",
"author": "Spencer Clem & ChatGPT",
"version": (2, 0),
"blender": (3, 0, 0),
"location": "View3D > Tools",
"description": "Import SketchUp .dae files as single objects with organized extras.",
"category": "Import-Export",
}
import bpy
import os
from bpy.props import StringProperty, PointerProperty
from bpy.types import Operator, Panel, PropertyGroup
class SketchUpDAEImportProperties(PropertyGroup):
dae_directory: StringProperty(
name="DAE Directory",
description="Directory containing the .dae files",
default="",
subtype="DIR_PATH",
)
class SketchUpDAEImportOperator(Operator):
bl_idname = "import.sketchup_dae_import"
bl_label = "Import .dae Files"
bl_description = "Import and process SketchUp .dae files as single objects"
bl_options = {"REGISTER", "UNDO"}
def execute(self, context):
props = context.scene.sketchup_dae_import_props
dae_directory = bpy.path.abspath(props.dae_directory) # Resolve path to absolute
# Validate directory
if not os.path.isdir(dae_directory):
self.report({"ERROR"}, "Invalid directory path")
return {"CANCELLED"}
# Get list of .dae files in the directory
dae_files = [f for f in os.listdir(dae_directory) if f.lower().endswith(".dae")]
if not dae_files:
self.report({"WARNING"}, "No .dae files found in the directory")
return {"CANCELLED"}
# Ensure the SketchUp Importer collection exists
main_collection = self.ensure_collection("SketchUp Importer")
extras_collection = self.ensure_collection("Extras", parent_collection=main_collection)
# Process each .dae file
for dae_file in dae_files:
file_path = os.path.join(dae_directory, dae_file)
# Track objects before import
existing_objects = set(bpy.data.objects)
# Import the .dae file
bpy.ops.wm.collada_import(filepath=file_path)
# Identify newly imported objects
imported_objects = [obj for obj in bpy.data.objects if obj not in existing_objects]
# Remove cameras and organize objects
remaining_objects = []
for obj in imported_objects:
if obj.type == "CAMERA":
bpy.data.objects.remove(obj, do_unlink=True) # Delete cameras
elif obj.type in {"CURVE", "EMPTY"}:
# Ensure objects are unlinked from all other collections
self.unlink_from_other_collections(obj)
extras_collection.objects.link(obj) # Move to Extras collection
else:
# Collect objects to be joined into geometry
remaining_objects.append(obj)
# Join remaining geometry into a single object
if remaining_objects:
for obj in remaining_objects:
obj.select_set(True)
bpy.context.view_layer.objects.active = remaining_objects[0]
bpy.ops.object.join()
# Rename the joined object to the .dae file name (without extension)
joined_object = bpy.context.view_layer.objects.active
joined_object.name = os.path.splitext(dae_file)[0]
# Ensure objects are unlinked from all other collections
self.unlink_from_other_collections(joined_object)
# Link the joined object to the SketchUp Importer collection
main_collection.objects.link(joined_object)
self.report({"INFO"}, "All .dae files processed successfully!")
return {"FINISHED"}
@staticmethod
def ensure_collection(name, parent_collection=None):
"""Ensure a collection with the given name exists. Optionally, link to a parent collection."""
if name not in bpy.data.collections:
collection = bpy.data.collections.new(name)
if parent_collection:
parent_collection.children.link(collection)
else:
bpy.context.scene.collection.children.link(collection)
return collection
return bpy.data.collections[name]
@staticmethod
def unlink_from_other_collections(obj):
"""Unlink the object from all collections it belongs to."""
for coll in obj.users_collection:
coll.objects.unlink(obj)
class SketchUpDAEImportPanel(Panel):
bl_idname = "VIEW3D_PT_sketchup_dae_import"
bl_label = "SketchUp .dae Importer"
bl_space_type = "VIEW_3D"
bl_region_type = "UI"
bl_category = "SketchUp Import"
def draw(self, context):
layout = self.layout
props = context.scene.sketchup_dae_import_props
layout.prop(props, "dae_directory")
# Add intended use description with wrapped text
box = layout.box()
box.label(text="Intended Use:", icon="INFO")
box.label(text="This addon is designed for use with a SketchUp Ruby export")
box.label(text="script that exports each tag as a separate .dae file. It")
box.label(text="enables the batch import of these files into Blender.")
# Add usage instructions with wrapped text
box = layout.box()
box.label(text="How to Use:", icon="INFO")
box.label(text="1. Select a folder containing .dae files.")
box.label(text="2. Click 'Import .dae Files'.")
box.label(text="3. Each .dae file is imported as a single object.")
box.label(text="4. Cameras are deleted.")
box.label(text="5. Curves and empties are moved to the 'Extras'")
box.label(text=" collection.")
box.label(text="6. All imports are organized under 'SketchUp Importer'.")
# Add the Import button
layout.operator("import.sketchup_dae_import", text="Import .dae Files")
# Add a link to your website below the button
layout.separator()
layout.label(text="For more scripts and tools, visit:")
layout.label(text="https://www.spencerclem.com/blog-posts-scripts", icon="URL")
classes = [SketchUpDAEImportProperties, SketchUpDAEImportOperator, SketchUpDAEImportPanel]
def register():
for cls in classes:
bpy.utils.register_class(cls)
bpy.types.Scene.sketchup_dae_import_props = PointerProperty(type=SketchUpDAEImportProperties)
def unregister():
for cls in classes:
bpy.utils.unregister_class(cls)
del bpy.types.Scene.sketchup_dae_import_props
if __name__ == "__main__":
register()
Description
The SketchUp .dae Importer is a powerful add-on for Blender users working with .dae
(Collada) files exported from SketchUp. It is specifically designed to complement a SketchUp Ruby export script that exports each tag as its own .dae
file, enabling efficient batch import into Blender for further processing.
Key Features:
Batch Import:The add-on allows users to select a folder containing multiple
.dae
files and import them all at once, eliminating the need for repetitive manual imports.
Object Consolidation:For each
.dae
file, all geometry is automatically joined into a single object. This ensures a clean and organized Outliner while maintaining the name of the original.dae
file for easy identification.
Extras Handling:Non-geometry objects like curves and empties are moved into a dedicated collection named Extras. This keeps the workspace tidy and separates non-essential elements from the main geometry.
Camera Removal:Any cameras included in the
.dae
files are automatically deleted during the import process, preventing unnecessary clutter.
Collection Organization:All imported objects are grouped under a master collection called SketchUp Importer. This collection is created automatically if it doesn’t already exist, providing a centralized location for all imported files.
Blender Compatibility:The add-on is compatible with Blender 4.2.0 and later, ensuring smooth integration with the latest features and improvements in Blender.
How It Works:
Folder Selection:The user selects a directory containing
.dae
files via the add-on’s UI in Blender.
Batch Import Process:
The add-on loops through all.dae
files in the selected folder and performs the following actions for each file:Imports the.dae
file into Blender.
Identifies all newly imported objects.
Object Cleanup:
The add-on processes imported objects as follows:Geometry: All imported geometry is joined into a single object and named after the original.dae
file.
Curves and Empties: Non-geometry objects are moved into the Extras collection.
Cameras: Any imported cameras are deleted.
Organizing Collections:The main geometry object is added to the SketchUp Importer collection.
If the SketchUp Importer or Extras collections don’t exist, the add-on creates them automatically.
Final Output:
After processing all files, the Outliner contains a clean hierarchy:
SketchUp Importer (master collection)Individual geometry objects (one per.dae
file)
Extras collection (containing curves and empties)
Enhanced Workflow:The add-on ensures a streamlined pipeline, making it easier to manage large projects with multiple tags and layers exported from SketchUp.
Intended Use:
This add-on is tailored for users who rely on SketchUp’s Ruby export script to generate .dae
files for each tag or layer. By automating the import and organization process in Blender, the add-on saves time, reduces errors, and improves efficiency for architects, designers, and 3D artists.
Use Case Example:
In SketchUp, a user organizes their model into layers or tags (e.g., walls, doors, windows).
Using the Ruby export script, they export each tag as a
.dae
file.In Blender, they use the SketchUp .dae Importer add-on to batch import these files, creating a structured and clean workspace with all objects consolidated and unnecessary elements removed.
Why It’s Useful:
Saves significant time for users importing multiple
.dae
files.Ensures a clean, organized Outliner with minimal manual cleanup.
Complements SketchUp’s layer/tag system for efficient data transfer into Blender.