Delete All Hidden Geometry (Including Hidden Tags)
This script permanently deletes all hidden geometry in a SketchUp model, including geometry on hidden tags and within nested groups or components.
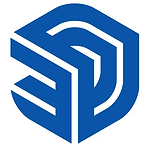
Broken button... I'll fix it eventually...
require 'set'
# Function to delete all hidden entities recursively, including those in groups and components
def delete_hidden_entities(entities, processed = Set.new)
entities.each do |entity|
# Skip already processed entities to avoid infinite loops
next if processed.include?(entity)
processed.add(entity)
# Delete the entity if it's hidden
if entity.respond_to?(:hidden?) && entity.hidden?
entity.erase! # Permanently delete the entity
next
end
# Recursively process groups and components
if entity.is_a?(Sketchup::Group) || entity.is_a?(Sketchup::ComponentInstance)
delete_hidden_entities(entity.definition.entities, processed)
end
end
end
# Main script execution
model = Sketchup.active_model
if model.nil?
UI.messagebox("No active SketchUp model found. Please open a model and try again.", MB_OK)
else
# Start operation to improve performance
model.start_operation("Delete Hidden Geometry", true)
# Make all tags visible to access hidden geometry on hidden tags
layers = model.layers
layers.each { |layer| layer.visible = true }
# Delete all hidden entities in the model
delete_hidden_entities(model.entities)
# Commit operation
model.commit_operation
# Notify user
UI.messagebox("All hidden geometry has been successfully deleted, including geometry on hidden tags!", MB_OK)
end
Description
What This Script Does
Checks Visibility of All Tags:Sets all tags to visible so that hidden geometry on hidden tags can be processed.
Deletes Hidden Geometry:Recursively iterates through all entities in the model and deletes any entity that is hidden.
Handles Nested Entities:Processes nested groups and components to ensure no hidden geometry is missed.
Steps to Use
Copy and paste this script into SketchUp’s Ruby Console or save it as a
.rb
file.Run the script in your SketchUp model.
The script will delete all hidden geometry, including entities on hidden tags.
Caution
Permanent Deletion: This script will permanently delete all hidden geometry. Ensure that you save your model or make a backup before running the script.
Tags Are Made Visible: After running the script, all tags will remain visible.
Let me know if you need further tweaks!